Image Classification with PyTorch and Cleanlab#
This 5-minute quickstart tutorial demonstrates how to find potential label errors in image classification data. Here we use the MNIST dataset containing 70,000 images of handwritten digits from 0 to 9.
Overview of what we’ll do in this tutorial:
Build a simple PyTorch neural net and wrap it with skorch to make it scikit-learn compatible.
Use this model to compute out-of-sample predicted probabilities,
pred_probs
, via cross-validation.Compute a list of potential label errors with cleanlab’s
find_label_issues
method.
Quickstart
Already have a model
? Run cross-validation to get out-of-sample pred_probs
and then the code below to get label issue indices ranked by their inferred severity.
from cleanlab.filter import find_label_issues
ranked_label_issues = find_label_issues(
labels,
pred_probs,
return_indices_ranked_by="self_confidence",
)
1. Install and import required dependencies#
You can use pip
to install all packages required for this tutorial as follows:
!pip install matplotlib torch torchvision skorch
!pip install cleanlab
# Make sure to install the version corresponding to this tutorial
# E.g. if viewing master branch documentation:
# !pip install git+https://github.com/cleanlab/cleanlab.git
[2]:
import torch
from torch import nn
from sklearn.datasets import fetch_openml
from sklearn.model_selection import cross_val_predict
from sklearn.metrics import accuracy_score
from skorch import NeuralNetClassifier
2. Fetch and scale the MNIST dataset#
[4]:
mnist = fetch_openml("mnist_784") # Fetch the MNIST dataset
X = mnist.data.astype("float32").to_numpy() # 2D array (images are flattened into 1D)
X /= 255.0 # Scale the features to the [0, 1] range
X = X.reshape(len(X), 1, 28, 28) # reshape into [N, C, H, W] for PyTorch
labels = mnist.target.astype("int64").to_numpy() # 1D array of given labels
/opt/hostedtoolcache/Python/3.10.11/x64/lib/python3.10/site-packages/sklearn/datasets/_openml.py:968: FutureWarning: The default value of `parser` will change from `'liac-arff'` to `'auto'` in 1.4. You can set `parser='auto'` to silence this warning. Therefore, an `ImportError` will be raised from 1.4 if the dataset is dense and pandas is not installed. Note that the pandas parser may return different data types. See the Notes Section in fetch_openml's API doc for details.
warn(
Bringing Your Own Data (BYOD)?
Assign your data’s features to variable X
and its labels to variable labels
instead.
Your classes (and entries of labels
) should be represented as integer indices 0, 1, …, num_classes - 1. For example, if your dataset has 7 examples from 3 classes, labels
might be: np.array([2,0,0,1,2,0,1])
3. Define a classification model#
Here, we define a simple neural network with PyTorch.
[5]:
class ClassifierModule(nn.Module):
def __init__(self):
super().__init__()
self.cnn = nn.Sequential(
nn.Conv2d(1, 6, 3),
nn.ReLU(),
nn.BatchNorm2d(6),
nn.MaxPool2d(kernel_size=2, stride=2),
nn.Conv2d(6, 16, 3),
nn.ReLU(),
nn.BatchNorm2d(16),
nn.MaxPool2d(kernel_size=2, stride=2),
)
self.out = nn.Sequential(
nn.Flatten(),
nn.LazyLinear(128),
nn.ReLU(),
nn.Linear(128, 10),
nn.Softmax(dim=-1),
)
def forward(self, X):
X = self.cnn(X)
X = self.out(X)
return X
4. Ensure your classifier is scikit-learn compatible#
As some cleanlab features require scikit-learn compatibility, we adapt the above PyTorch neural net accordingly. skorch is a convenient package that helps with this. Alternatively, you can also easily wrap an arbitrary model to be scikit-learn compatible as demonstrated here.
[6]:
model_skorch = NeuralNetClassifier(ClassifierModule)
5. Compute out-of-sample predicted probabilities#
If we’d like cleanlab to identify potential label errors in the whole dataset and not just the training set, we can consider using the entire dataset when computing the out-of-sample predicted probabilities, pred_probs
, via cross-validation.
[7]:
num_crossval_folds = 3 # for efficiency; values like 5 or 10 will generally work better
pred_probs = cross_val_predict(
model_skorch,
X,
labels,
cv=num_crossval_folds,
method="predict_proba",
)
epoch train_loss valid_acc valid_loss dur
------- ------------ ----------- ------------ ------
1 0.6908 0.9139 0.3099 3.4316
2 0.2112 0.9412 0.2002 3.1783
3 0.1521 0.9516 0.1574 3.1724
4 0.1240 0.9594 0.1332 3.1816
5 0.1066 0.9633 0.1178 3.1970
6 0.0948 0.9660 0.1072 3.1340
7 0.0860 0.9682 0.0994 3.1801
8 0.0792 0.9708 0.0934 3.0898
9 0.0737 0.9725 0.0886 3.1161
10 0.0690 0.9736 0.0847 3.1384
epoch train_loss valid_acc valid_loss dur
------- ------------ ----------- ------------ ------
1 0.7043 0.9247 0.2786 3.2048
2 0.1907 0.9465 0.1817 3.1597
3 0.1355 0.9556 0.1477 3.1896
4 0.1100 0.9616 0.1289 3.1762
5 0.0943 0.9648 0.1166 3.1640
6 0.0834 0.9684 0.1079 3.1294
7 0.0751 0.9702 0.1014 3.1435
8 0.0687 0.9713 0.0963 3.1220
9 0.0634 0.9724 0.0921 3.0993
10 0.0589 0.9732 0.0887 3.1072
epoch train_loss valid_acc valid_loss dur
------- ------------ ----------- ------------ ------
1 0.7931 0.9112 0.3372 3.1508
2 0.2282 0.9486 0.1948 3.1500
3 0.1533 0.9592 0.1501 3.1771
4 0.1217 0.9641 0.1277 3.2656
5 0.1032 0.9678 0.1135 3.1925
6 0.0903 0.9701 0.1037 3.2295
7 0.0809 0.9729 0.0964 3.1623
8 0.0736 0.9747 0.0903 3.2574
9 0.0677 0.9761 0.0861 3.2684
10 0.0630 0.9766 0.0825 3.3102
An additional benefit of cross-validation is that it facilitates more reliable evaluation of our model than a single training/validation split.
[8]:
predicted_labels = pred_probs.argmax(axis=1)
acc = accuracy_score(labels, predicted_labels)
print(f"Cross-validated estimate of accuracy on held-out data: {acc}")
Cross-validated estimate of accuracy on held-out data: 0.9752428571428572
6. Use cleanlab to find label issues#
Based on the given labels and out-of-sample predicted probabilities, cleanlab can quickly help us identify label issues in our dataset. For a dataset with N examples from K classes, the labels should be a 1D array of length N and predicted probabilities should be a 2D (N x K) array. Here we request that the indices of the identified label issues be sorted by cleanlab’s self-confidence score, which measures the quality of each given label via the probability assigned to it in our model’s prediction.
[9]:
from cleanlab.filter import find_label_issues
ranked_label_issues = find_label_issues(
labels,
pred_probs,
return_indices_ranked_by="self_confidence",
)
print(f"Cleanlab found {len(ranked_label_issues)} label issues.")
print(f"Top 15 most likely label errors: \n {ranked_label_issues[:15]}")
Cleanlab found 143 label issues.
Top 15 most likely label errors:
[59915 24798 19124 53216 2720 59701 50340 7010 40976 16376 23824 44484
500 8729 31134]
ranked_label_issues
is a list of indices corresponding to examples that are worth inspecting more closely. To help visualize specific examples, we define a plot_examples
function (can skip these details).
See the implementation of plot_examples
(click to expand)
plot_examples
(click to expand)# Note: This pulldown content is for docs.cleanlab.ai, if running on local Jupyter or Colab, please ignore it.
import matplotlib.pyplot as plt
def plot_examples(id_iter, nrows=1, ncols=1):
for count, id in enumerate(id_iter):
plt.subplot(nrows, ncols, count + 1)
plt.imshow(X[id].reshape(28, 28), cmap="gray")
plt.title(f"id: {id} \n label: {y[id]}")
plt.axis("off")
plt.tight_layout(h_pad=2.0)
Let’s look at the top 15 examples cleanlab thinks are most likely to be incorrectly labeled. We can see a few label errors and odd edge cases. Feel free to change the values below to display more/fewer examples.
[11]:
plot_examples(ranked_label_issues[range(15)], 3, 5)
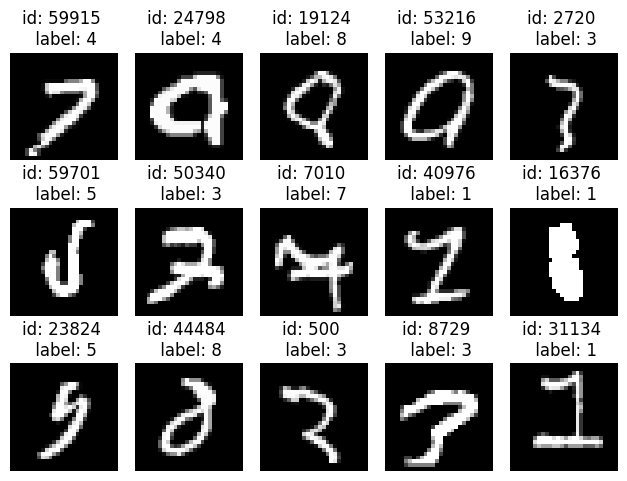
Let’s zoom into some specific examples from the above set:
Given label is 4 but looks more like a 7:
[12]:
plot_examples([59915])
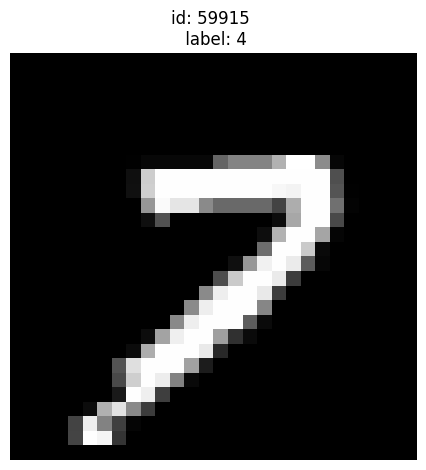
Given label is 4 but also looks like 9:
[13]:
plot_examples([24798])
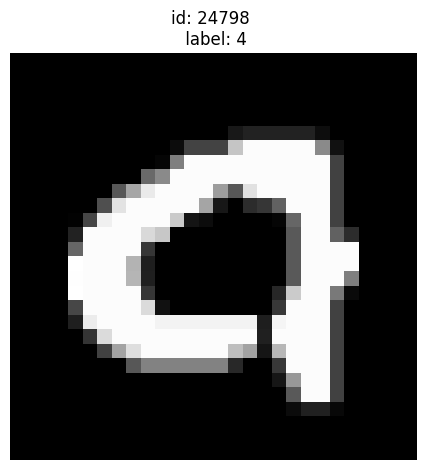
A very odd looking 5:
[14]:
plot_examples([59701])
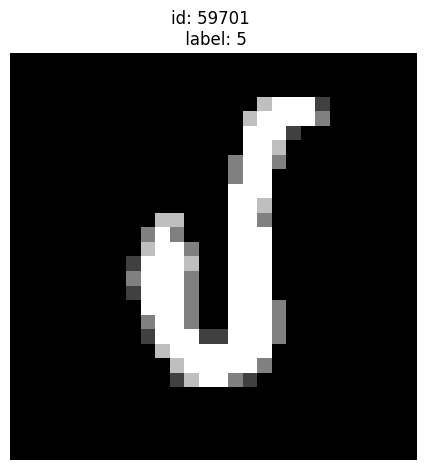
Given label is 3 but could be a 7:
[15]:
plot_examples([50340])
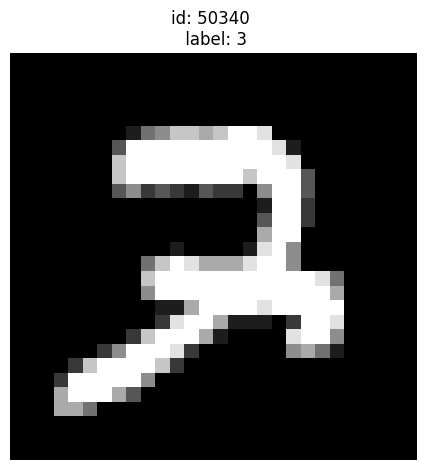
cleanlab has shortlisted the most likely label errors to speed up your data cleaning process. With this list, you can decide whether to fix label issues or prune some of these examples from the dataset.
You can see that even widely-used datasets like MNIST contain problematic labels. Never blindly trust your data! You should always check it for potential issues, many of which can be easily identified by cleanlab.